Google Play Games Services
A Cordova plugin that let's you interact with Google Play Games Services.
https://github.com/artberri/cordova-plugin-play-games-services
Stuck on a Cordova issue?
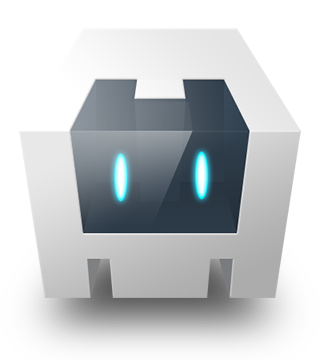
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionic’s experts offer premium advisory services for both community plugins and premier plugins.
Installation#
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-play-games-services $ npm install @ionic-native/google-play-games-services $ ionic cap sync
$ ionic cordova plugin add cordova-plugin-play-games-services $ npm install @ionic-native/google-play-games-services
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Learn More or if you're interested in an enterprise version of this plugin Contact Us
#
Supported Platforms- Android
#
Usage#
ReactLearn more about using Ionic Native components in React
#
Angularimport { GooglePlayGamesServices } from '@ionic-native/google-play-games-services/ngx';
constructor(private googlePlayGamesServices: GooglePlayGamesServices) { }
...
// Authenticate with Play Games Servicesthis.googlePlayGamesServices.auth() .then(() => console.log('Logged in to Play Games Services')) .catch(e) => console.log('Error logging in Play Games Services', e);
// Sign out of Play Games Services.this.googlePlayGamesServices.signOut() .then(() => console.log('Logged out of Play Games Services')) .catch(e => console.log('Error logging out of Play Games Services', e);
// Check auth status.this.googlePlayGamesServices.isSignedIn() .then((signedIn: SignedInResponse) => { if (signedIn.isSignedIn) { hideLoginButton(); } });
// Fetch currently authenticated user's data.this.googlePlayGamesServices.showPlayer().then((data: Player) => { console.log('Player data', data);});
// Submit a score.this.googlePlayGamesServices.submitScore({ score: 100, leaderboardId: 'SomeLeaderboardId'});
// Submit a score and wait for reponse.this.googlePlayGamesServices.submitScoreNow({ score: 100, leaderboardId: 'SomeLeaderboardId'}).then((data: SubmittedScoreData) => { console.log('Score related data', data);});
// Get the player score on a leaderboard.this.googlePlayGamesServices.getPlayerScore({ leaderboardId: 'SomeLeaderBoardId'}).then((data: PlayerScoreData) => { console.log('Player score', data);});
// Show the native leaderboards window.this.googlePlayGamesServices.showAllLeaderboards() .then(() => console.log('The leaderboard window is visible.'));
// Show a signle native leaderboard window.this.googlePlayGamesServices.showLeaderboard({ leaderboardId: 'SomeLeaderBoardId'}).then(() => console.log('The leaderboard window is visible.'));
// Unlock an achievement.this.googlePlayGamesServices.unlockAchievement({ achievementId: 'SomeAchievementId'}).then(() => console.log('Achievement sent'));
// Unlock an achievement and wait for response.this.googlePlayGamesServices.unlockAchievementNow({ achievementId: 'SomeAchievementId'}).then(() => console.log('Achievement unlocked'));
// Incremement an achievement.this.googlePlayGamesServices.incrementAchievement({ step: 1, achievementId: 'SomeAchievementId'}).then(() => console.log('Achievement increment sent'));
// Incremement an achievement and wait for response.this.googlePlayGamesServices.incrementAchievementNow({ step: 1, achievementId: 'SomeAchievementId'}).then(() => console.log('Achievement incremented'));
// Show the native achievements window.this.googlePlayGamesServices.showAchivements() .then(() => console.log('The achievements window is visible.'));