Class Kit
Plugin for using Apple's ClassKit framework.
Prerequisites: Only works with Xcode 9.4 and iOS 11.4. Your Provisioning Profile must include the ClassKit capability. Read more about how to Request ClassKit Resources (https://developer.apple.com/contact/classkit/) in here: https://developer.apple.com/documentation/classkit/enabling_classkit_in_your_app. Also note that you can’t test ClassKit behavior in Simulator because Schoolwork isn’t available in that environment.
https://github.com/sebastianbaar/cordova-plugin-classkit.git
Stuck on a Cordova issue?
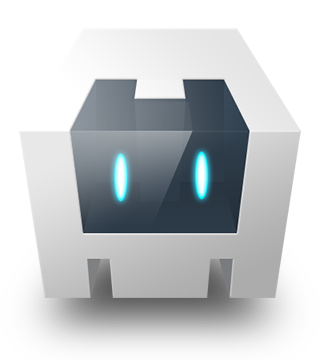
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionic’s experts offer premium advisory services for both community plugins and premier plugins.
Installation#
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-plugin-classkit $ npm install @ionic-native/class-kit $ ionic cap sync
$ ionic cordova plugin add cordova-plugin-classkit $ npm install @ionic-native/class-kit
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Learn More or if you're interested in an enterprise version of this plugin Contact Us
#
Supported Platforms- iOS
#
Usage#
ReactLearn more about using Ionic Native components in React
#
Angularimport { ClassKit, CCKContext, CCKBinaryItem, CCKQuantityItem, CCKScoreItem, CCKContextTopic, CCKContextType, CCKBinaryType } from '@ionic-native/class-kit/ngx';
// Init contexts defined in XML file 'CCK-contexts.xml'constructor( ..., private classKit: ClassKit) { platform.ready().then(() => { classKit.initContextsFromXml("classkitplugin://") .then(() => console.log("success")) .catch(e => console.log("error: ", e)); });}
...
// Init context with identifier pathconst context: CCKContext = { identifierPath: ["parent_title_one", "child_one", "child_one_correct_quiz"], title: "child one correct quiz", type: CCKContextType.exercise, topic: CCKContextTopic.science, displayOrder: 0};
this.classKit.addContext("classkitplugin://", context) .then(() => console.log("success")) .catch(e => console.log("error: ", e));
// Remove all contextsthis.classKit.removeContexts() .then(() => console.log("success")) .catch(e => console.log("error: ", e));
// Remove context with identifier paththis.classKit.removeContext(["parent_title_one", "child_one", "child_one_correct_quiz"]) .then(() => console.log("success")) .catch(e => console.log("error: ", e));
// Begin a new activity or restart an activity for a given contextthis.classKit.beginActivity(["parent_title_one", "child_two", "child_two_quiz"], false) .then(() => console.log("success")) .catch(e => console.log("error: ", e));
// Adds a progress range to the active given activitythis.classKit.setProgressRange(0, 0.66) .then(() => console.log("success")) .catch(e => console.log("error: ", e));
// Adds a progress to the active given activitythis.classKit.setProgress(0.66) .then(() => console.log("success")) .catch(e => console.log("error: ", e));
// Adds activity information that is true or false, pass or fail, yes or noconst binaryItem: CCKBinaryItem = { identifier: "child_two_quiz_IDENTIFIER_1", title: "CHILD TWO QUIZ 1", type: CCKBinaryType.trueFalse, isCorrect: isCorrect, isPrimaryActivityItem: false};
this.classKit.setBinaryItem(binaryItem) .then(() => console.log("success")) .catch(e => console.log("error: ", e));
// Adds activity information that signifies a score out of a possible maximumconst scoreItem: CCKScoreItem = { identifier: "total_score", title: "Total Score :-)", score: 0.66, maxScore: 1.0, isPrimaryActivityItem: true};
this.classKit.setScoreItem(scoreItem) .then(() => console.log("success")) .catch(e => console.log("error: ", e));
// Activity information that signifies a quantityconst quantityItem: CCKQuantityItem = { identifier: "quantity_item_hints", title: "Hints", quantity: 12, isPrimaryActivityItem: false};
this.classKit.setQuantityItem(quantityItem) .then(() => console.log("success")) .catch(e => console.log("error: ", e));