WheelSelector Plugin
Native wheel selector for Cordova (Android/iOS).
https://github.com/jasonmamy/cordova-wheel-selector-plugin
Stuck on a Cordova issue?
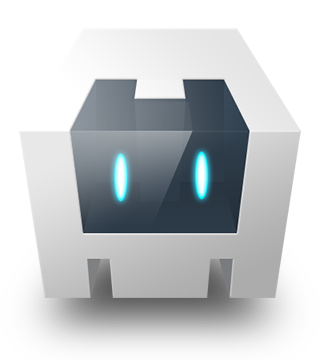
If you're building a serious project, you can't afford to spend hours troubleshooting. Ionic’s experts offer premium advisory services for both community plugins and premier plugins.
Installation#
- Capacitor
- Cordova
- Enterprise
$ npm install cordova-wheel-selector-plugin $ npm install @ionic-native/wheel-selector $ ionic cap sync
$ ionic cordova plugin add cordova-wheel-selector-plugin $ npm install @ionic-native/wheel-selector
Ionic Enterprise comes with fully supported and maintained plugins from the Ionic Team. Learn More or if you're interested in an enterprise version of this plugin Contact Us
#
Supported Platforms- Android
- iOS
#
Usage#
ReactLearn more about using Ionic Native components in React
#
Angularimport { WheelSelector } from '@ionic-native/wheel-selector/ngx';
constructor(private selector: WheelSelector) { }
...
const jsonData = { numbers: [ { description: "1" }, { description: "2" }, { description: "3" } ], fruits: [ { description: "Apple" }, { description: "Banana" }, { description: "Tangerine" } ], firstNames: [ { name: "Fred", id: '1' }, { name: "Jane", id: '2' }, { name: "Bob", id: '3' }, { name: "Earl", id: '4' }, { name: "Eunice", id: '5' } ], lastNames: [ { name: "Johnson", id: '100' }, { name: "Doe", id: '101' }, { name: "Kinishiwa", id: '102' }, { name: "Gordon", id: '103' }, { name: "Smith", id: '104' } ]}
...
// basic number selection, index is always returned in the result selectANumber() { this.selector.show({ title: "How Many?", items: [ this.jsonData.numbers ], }).then( result => { console.log(result[0].description + ' at index: ' + result[0].index); }, err => console.log('Error: ', err) ); }
...
// basic selection, setting initial displayed default values: '3' 'Banana' selectFruit() { this.selector.show({ title: "How Much?", items: [ this.jsonData.numbers, this.jsonData.fruits ], positiveButtonText: "Ok", negativeButtonText: "Nope", defaultItems: [ {index:0, value: this.jsonData.numbers[2].description}, {index: 1, value: this.jsonData.fruits[3].description} ] }).then( result => { console.log(result[0].description + ' ' + result[1].description); }, err => console.log('Error: ' + JSON.stringify(err)) ); }
...
// more complex as overrides which key to display // then retrieve properties from original data selectNamesUsingDisplayKey() { this.selector.show({ title: "Who?", items: [ this.jsonData.firstNames, this.jsonData.lastNames ], displayKey: 'name', defaultItems: [ {index:0, value: this.jsonData.firstNames[2].name}, {index: 0, value: this.jsonData.lastNames[3].name} ] }).then( result => { console.log(result[0].name + ' (id= ' + this.jsonData.firstNames[result[0].index].id + '), ' + result[1].name + ' (id=' + this.jsonData.lastNames[result[1].index].id + ')'); }, err => console.log('Error: ' + JSON.stringify(err)) ); }